Fetch v/s Axios
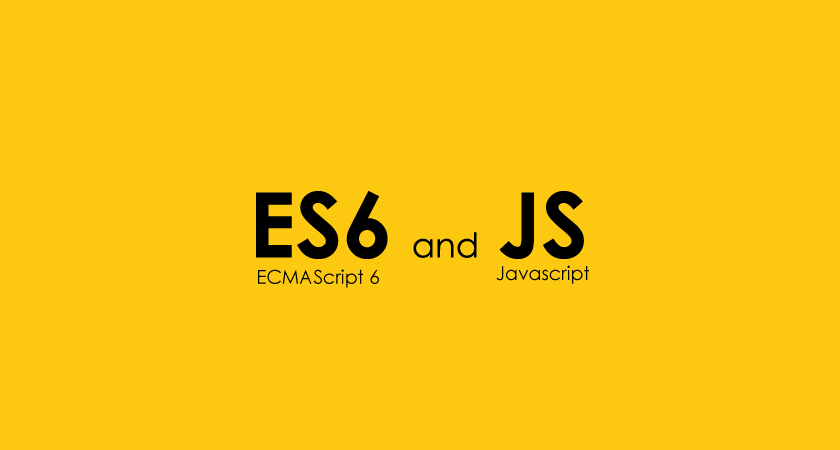
Communicating with an API is a very common aspect of developing any web-based project and all the developers must know how to fetch some stuff from an API and play around with it as per the needs. I always had this question in mind that why do we need a separate library such as Axios for making HTTP requests when we have something such as the Fetch API in ES6 right out of the box, available natively. And so I digged in a bit and found something worth sharing. In this short blog post, I will try to throw some light on the same.
There are some shortcomings in using the native fetch() method and some things that aren't ideal and so we need other options such as axios.
Axios is a Javascript library used to make http requests from node.js or XMLHttpRequests from the browser and it supports the Promise API that is native to JS ES6. Another feature that it has over .fetch() is that it performs automatic transforms of JSON data.
If you use .fetch() there is a two-step process when handing JSON data. The first is to make the actual request and then the second is to call the .json() method on the response.
Here is a simple example using the JSON Placeholder API. I set the url as a variable and then pass it to fetch and set the .then() callback to console the data that gets returned from the request. (as we get back a promise using the Fetch API).
That’s great, but this is not the data you are looking for. That is the response from the server letting you know that your request went through just fine. Great, but you can’t do much with that.
To get to that data, you have to pass it to .json() first and then you can see it.
Let’s see how this is handled with Axios.
The first step in using Axios is installing Axios. You can use npm if you want to run axios in node or a cdn if you want to run it in your browser.
Then, in my console, I assign the
url
variable and then pass it to the axios.get()
method.So by using axios you can cut out the middle step of passing the results of the http request to the .json() method. Axios just returns the data object you would expect.
The second issue is how .fetch() handles error responses. Logically you would think that if .fetch() gets an error it would enter the .catch() block and return anything there, right? Not necessarily. Here is an example.
I have altered my
url
variable from the previous examples so that it is now incorrect. I would expect a 400 error at this point and for my .fetch() to go into the .catch() block but this is not what happens instead.I get the 400 response code but, as you can see by the ‘GOOD’ string in the console, the .then() block was executed. How does Axios handle this? ( I leave this as a homework for you, try it out yourself and see the result). The way you would probably expect. You get any kind of error with the http request and the .catch() block is executed.
The ‘BAD’ string is there and the error is logged to the console.
The .fetch() method is a great step in the right direction of getting http requests native in ES6, but just know that if you use it there are a couple of gotchas that might be better handled by third-party libraries like Axios. Its always good to use libraries such as Axios when its available to ease out the development process and avoid some minor housekeeping stuff.
I hope this was helpful and added to your knowledge base a bit. Thanks for reading. New post will be up soon. Till then, keep learning, keep developing!
Comments
Post a Comment